少し修正したので貼っておこー
機能追加
- タスクトレイのアイコンに現在の仮想デスクトップの番号を表示
- タスクトレイのアイコンのメニューからデスクトップを移動
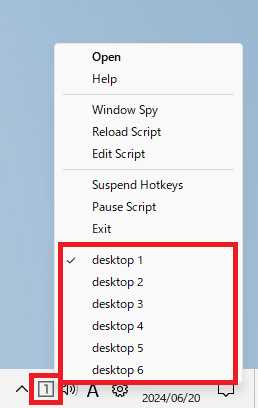
作ったもの
「タスクビュー」のソースファイル
#Requires AutoHotkey v2.0+
#SingleInstance Force
; ----------------------------------------
; define
; ----------------------------------------
PREV := "Prev"
NEXT := "Next"
OK := True
NG := False
BASE_DIR := "C:\tool\taskview\"
VDA_PATH := BASE_DIR . "VirtualDesktopAccessor.dll"
TRAYICON_PATH := BASE_DIR . "images\"
hVirtualDesktopAccessor := DllCall("LoadLibrary", "Str", VDA_PATH, "Ptr")
GetDesktopCountProc := DllCall("GetProcAddress", "Ptr", hVirtualDesktopAccessor, "AStr", "GetDesktopCount", "Ptr")
GoToDesktopNumberProc := DllCall("GetProcAddress", "Ptr", hVirtualDesktopAccessor, "AStr", "GoToDesktopNumber", "Ptr")
GetCurrentDesktopNumberProc := DllCall("GetProcAddress", "Ptr", hVirtualDesktopAccessor, "AStr", "GetCurrentDesktopNumber", "Ptr")
MoveWindowToDesktopNumberProc := DllCall("GetProcAddress", "Ptr", hVirtualDesktopAccessor, "AStr", "MoveWindowToDesktopNumber", "Ptr")
; ----------------------------------------
; helper function
; ----------------------------------------
GetDesktopCount() {
count := DllCall(GetDesktopCountProc, "Int")
return count
}
GoToDesktopNumber(number) {
if (number > GetDesktopCount())
return
; Win + 左右キーのアニメーションに操作感を合わせるため、
; 移動前後に0.5秒の待ち時間を設定している(機能的には不要)
Sleep 500
DllCall(GoToDesktopNumberProc, "Int", number - 1)
Sleep 500
}
GetCurrentDesktopNumber() {
number := DllCall(GetCurrentDesktopNumberProc, "Int")
number++ ; デスクトップ番号を1オリジンで指定するため + 1
return number
}
MoveWindowToDesktopNumber(number) {
WinWaitActive WinExist("A")
retry := 0
Loop
try {
activeHwnd := WinGetID("A")
number-- ; dllには0オリジンで渡すため - 1
DllCall(MoveWindowToDesktopNumberProc, "Ptr", activeHwnd, "Int", number, "Int")
result := OK
break
} catch {
Sleep 250
result := NG
retry++
}
until retry == 10
return result
}
moveWindow(desktop) {
current := GetCurrentDesktopNumber()
max := GetDesktopCount()
if (desktop == PREV) {
if (current == 1) {
result := MoveWindowToDesktopNumber(max)
} else {
result := MoveWindowToDesktopNumber(current - 1)
}
} else if (desktop == NEXT) {
if (current == max) {
result := MoveWindowToDesktopNumber(1)
} else {
result := MoveWindowToDesktopNumber(current + 1)
}
} else {
result := MoveWindowToDesktopNumber(desktop)
}
return result
}
moveDesktop(desktop) {
current := GetCurrentDesktopNumber()
max := GetDesktopCount()
if (desktop == PREV) {
if (current == 1) {
GoToDesktopNumber(max)
} else {
Send "^#{Left}"
}
KeyWait "Left"
} else if (desktop == NEXT) {
if (current == max) {
GoToDesktopNumber(1)
} else {
Send "^#{Right}"
}
KeyWait "Right"
} else {
GoToDesktopNumber(desktop)
}
}
moveWindowMoveDesktop(desktop) {
result := moveWindow(desktop)
if (result == OK)
moveDesktop(desktop)
}
; ----------------------------------------
; tasktray
; ----------------------------------------
; メニュー追加
A_TrayMenu.Add()
num := 1
Loop GetDesktopCount() {
A_TrayMenu.Add("desktop " . num, MenuHandler)
num++
}
MenuHandler(itemName, itemPos, myMenu) {
itemCount := DllCall("GetMenuItemCount", "ptr", A_TrayMenu.Handle)
startNum := itemCount - GetDesktopCount()
newNum := itemPos - startNum
moveDesktop(newNum)
}
; タスクトレイ更新
iconNum := 1
Loop {
currNum := GetCurrentDesktopNumber()
if (iconNum != currNum) {
try {
; アイコン
TraySetIcon(TRAYICON_PATH . currNum . ".png")
; メニュー
num := 1
Loop GetDesktopCount() {
A_TrayMenu.Uncheck("desktop " . num)
num++
}
A_TrayMenu.Check("desktop " . currNum)
}
iconNum := currNum
}
Sleep 1000
}
; ----------------------------------------
; main
; ----------------------------------------
; アクティブ画面移動
^+Left:: moveWindow(PREV)
^+Right:: moveWindow(NEXT)
^+F1:: moveWindow(1)
^+F2:: moveWindow(2)
^+F3:: moveWindow(3)
^+F4:: moveWindow(4)
^+F5:: moveWindow(5)
^+F6:: moveWindow(6)
; デスクトップ移動
^#Left:: moveDesktop(PREV)
^#Right:: moveDesktop(NEXT)
^#F1:: moveDesktop(1)
^#F2:: moveDesktop(2)
^#F3:: moveDesktop(3)
^#F4:: moveDesktop(4)
^#F5:: moveDesktop(5)
^#F6:: moveDesktop(6)
; アクティブ画面/デスクトップ移動
^#+Left:: moveWindowMoveDesktop(PREV)
^#+Right:: moveWindowMoveDesktop(NEXT)
^#+F1:: moveWindowMoveDesktop(1)
^#+F2:: moveWindowMoveDesktop(2)
^#+F3:: moveWindowMoveDesktop(3)
^#+F4:: moveWindowMoveDesktop(4)
^#+F5:: moveWindowMoveDesktop(5)
^#+F6:: moveWindowMoveDesktop(6)